php+mysql 实现简单登录页面(带验证码功能)
实现目标
1、登录界面
用户名输入框
密码输入框
验证码输入框
提交按钮
2、数据库新建一个用户表admin:
设置字段:
- user
- password
在数据库中手动添加一条用户记录
3、登录验证文件
- 判断登录页面提交的用户名、密码和数据库中的用户名和密码比对,
- 验证码和session中的验证码比对,
- 判断提示登录成功或用户名错误、验证码错
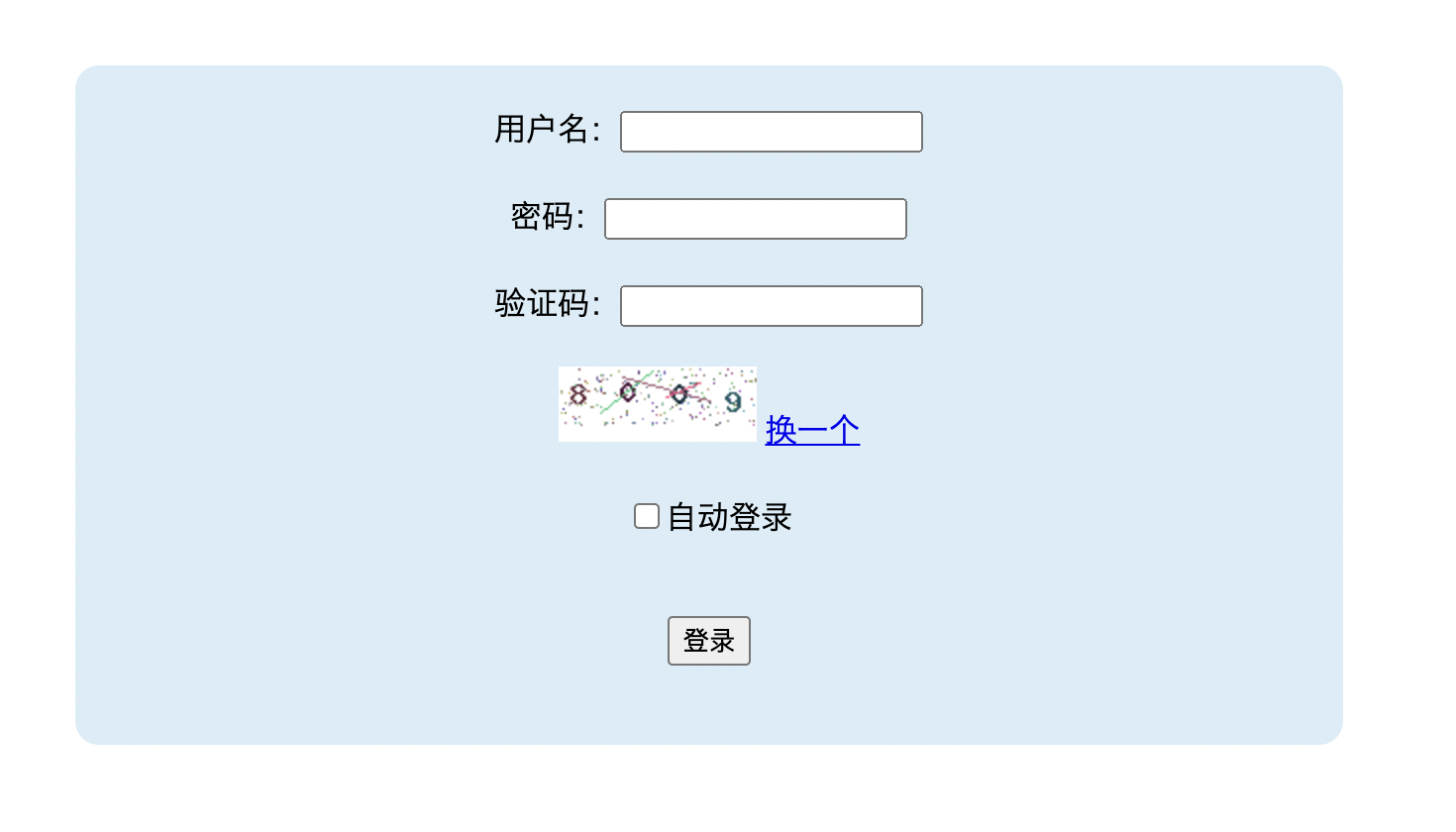
登录界面
index.html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="utf-8">
<title>登录</title>
<style>
body{
font: 16px arial,'Microsoft Yahei','Hiragino Sans GB',sans-serif;
}
h1{
margin: 0;
color:#3a87ad;
font-size: 26px;
}
.content{
width: 45%;
margin: 0 auto;
}
.content >div{
margin-top: 200px;
padding: 20px;
background: #d9edf7;
border-radius: 12px;
}
.content dl{
color: #2d6a88;
line-height: 40px;
}
.content div div {
padding-bottom: 20px;
text-align:center;
}
</style>
</head>
<body>
<div class="content">
<div>
<form method="post" action="login.php">
<div>用户名:<input type="text" name="username"></div>
<div>密码:<input type="password" name="password"></div>
<div>验证码:<input type="text" name="verifycode" class="captcha"></div>
<div>
<img id="captcha_img" src="captcha.php?r=<?php echo rand();?>" alt="验证码">
<label><a href="javascript:void(0)" rel="external nofollow" onclick="document.getElementById('captcha_img').src='captcha.php?r='+Math.random()">换一个</a> </label>
</div>
<div><input type="checkbox" name="autologin[]" value="1"/>自动登录</div><br>
<div><button type="submit">登录</button></form></div>
</form>
</div>
</div>
</body>
</html>
验证码
captcha.php
<?php
/*PHP实现验证码*/
session_start();//开启会话
//创建画布
$image=imagecreatetruecolor(100,38);
//背景颜色
$bgcolor=imagecolorallocate($image,255,255,255);
imagefill($image,0,0,$bgcolor);
$captch_code='';//存储验证码
//随机选取4个数字
for($i=0;$i<4;$i++){
$fontsize=10; //
$fontcolor=imagecolorallocate($image,rand(0,120),rand(0,120),rand(0,120));//随机颜色
$fontcontent=rand(0,9);
$captch_code.=$fontcontent;
$x=($i*100/4)+rand(5,10); //随机坐标
$y=rand(5,10);
imagestring($image,$fontsize,$x,$y,$fontcontent,$fontcolor);
}
/*//字母和数字混合验证码
for($i=0;$i<4;$i++) {
$fontsize = 10; //
$fontcolor = imagecolorallocate($image, rand(0, 120), rand(0, 120), rand(0, 120));//??????
$data = 'abcdefghijklmnopqrstuvwxyz1234567890'; //数据字典
$fontcontent = substr($data, rand(0, strlen($data)), 1);
$captch_code.=$fontcontent;
$x = ($i * 100 / 4) + rand(5, 10);
$y = rand(5, 10);
imagestring($image, $fontsize, $x, $y, $fontcontent, $fontcolor);
}*/
$_SESSION['code']=$captch_code;
//增加干扰点
for($i=0;$i<200;$i++){
$pointcolor=imagecolorallocate($image,rand(50,200),rand(50,200),rand(50,200));
imagesetpixel($image,rand(1,99),rand(1,29),$pointcolor);//
}
//增加干扰线
for($i=0;$i<3;$i++){
$linecolor=imagecolorallocate($image,rand(80,280),rand(80,220),rand(80,220));
imageline($image,rand(1,99),rand(1,29),rand(1,99),rand(1,29),$linecolor);
}
//输出格式
header('content-type:image.png');
imagepng($image);
//销毁图片
imagedestroy($image);
?>
登录验证
login.php
<?php
header("Content-type:text/html;charset=UTF-8");
require "conn.php"; //访问数据库
session_start(); //开启会话一获取到服务器端验证码
$username=$_POST['username'];
$password=$_POST['password'];
$autologin=isset($_POST['autologin'])?1:0; //获取是否选择了自动登录
$verifycode=$_POST['verifycode'];
$code=$_SESSION['code']; //获取服务器生成的验证码
/*
* 首先进行判空操作,通过后进行验证码验证,通过后再进行数据库验证。
* 手机号码和邮箱验证可根据需要自行添加
* */
if(checkEmpty($username,$password,$verifycode)){
if(checkVerifycode($verifycode,$code)){
if(checkUser($username,$password)){
$_SESSION['username']=$username; //保存此时登录成功的用户名
if($autologin==1){ //如果用户勾选了自动登录就把用户名和加了密的密码放到cookie里面
setcookie("username",$username,time()+3600*24*3); //有效期设置为3天
setcookie("password",md5($password),time()+3600*24*3);
}
else{
setcookie("username","",time()-1); //如果没有选择自动登录就清空cookie
setcookie("password","",time()-1);
}
header("location: home.php "); //全部验证都通过之后跳转到首页
}
}
}
//方法:判断是否为空
function checkEmpty($username,$password,$verifycode){
if($username==null||$password==null){
echo '<html><head><Script Language="JavaScript">alert("用户名或密码为空");</Script></head></html>' . "<meta http-equiv=\"refresh\" content=\"0;url=index.html\">";
}
else{
if($verifycode==null){
echo '<html><head><Script Language="JavaScript">alert("验证码为空");</Script></head></html>' . "<meta http-equiv=\"refresh\" content=\"0;url=index.html\">";
}
else{
return true;
}
}
}
//方法:检查验证码是否正确
function checkVerifycode($verifycode,$code){
if($verifycode==$code){
return true;
}
else{
echo '<html><head><Script Language="JavaScript">alert("验证码错误");</Script></head></html>' . "<meta http-equiv=\"refresh\" content=\"0;url=index.html\">";
}
}
//方法:查询用户是否在数据库中
function checkUser($username,$password){
$conn=new Mysql();
$sql="select * from user where username='$username' and password='$password';";
$result=$conn->sql($sql);
if($result){
return true;
}
else{
echo '<html><head><Script Language="JavaScript">alert("用户不存在");</Script></head></html>' . "<meta http-equiv=\"refresh\" content=\"0;url=index.html\">";
}
$conn->close();//关闭数据库
}
//方法:手机格式验证
function checkPhoneNum($phonenumber){
$preg="/^1[34578]{1}\d{9}$/";
if(preg_match($preg,$phonenumber)){
return ture; //验证通过
}else{
echo '<html><head><Script Language="JavaScript">alert("手机号码格式有误");</Script></head></html>' . "<meta http-equiv=\"refresh\" content=\"0;url=index.html\">";//手机号码格式不对
}
}
//方法:邮箱格式验证
function checkEmail($email){
$preg = '/^(\w{1,25})@(\w{1,16})(\.(\w{1,4})){1,3}$/';
if(preg_match($preg, $email)){
return true;
}else{
echo '<html><head><Script Language="JavaScript">alert("邮箱格式有误");</Script></head></html>' . "<meta http-equiv=\"refresh\" content=\"0;url=index.html\">";
}
}
数据库连接
conn.php
<?php
class Mysql{
private static $host="localhost";
private static $user="root";
private static $password="23102310";
private static $dbName="chat"; //数据库名
private static $charset="utf8"; //字符编码
private static $port="3306"; //端口号
private $conn=null;
function __construct(){
$this->conn=new mysqli(self::$host,self::$user,self::$password,self::$dbName,self::$port);
if(!$this->conn)
{
die("数据库连接失败!".$this->conn->connect_error);
}else{
echo "连接成功!";
}
$this->conn->query("set names ".self::$charset);
}
//执行sql语句
function sql($sql){
$res=$this->conn->query($sql);
if(!$res)
{
echo "数据操作失败";
}
else
{
if($this->conn->affected_rows>0)
{
return $res;
}
else
{
echo "0行数据受影响!";
}
}
}
//返回受影响数据行数
function getResultNum($sql){
$res=$this->conn->query($sql);
return mysqli_num_rows($res);
}
//关闭数据库
public function close()
{
@mysqli_close($this->conn);
}
}
$conn=new Mysql();
//关闭数据库
$conn->close();
?>
登录成功页面
home.php
<?php
// 开启Session
session_start();
// 首先判断Cookie是否有记住了用户信息
if(empty($_COOKIE['username'])&&empty($_COOKIE['password'])){
if(isset($_SESSION['username']))
echo "登录成功,欢迎您".$_SESSION['username']."<a href='logout.php'>退出登录</a>";
else
echo "你还没有登录,<a href='index.html'>请登录</a>";
}
else
echo "登录成功,欢迎您:".$_COOKIE['username']."<a href='logout.php'>退出登录</a>";
?>
数据库
创建表
CREATE TABLE `user` (
`username` varchar(20) NOT NULL PRIMARY KEY,
`password` varchar(20)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
向表中添加数据
INSERT INTO `user` (`username`, `password`) VALUES
('admin', 'admin');
创建了一个用户名为admin,密码为admin的数据